Read the node properties¶
Problem Description¶
In this example we will read the nodes data from the database.
Following values are extracted:
NR
(node number)INR
(internal node number)KFIX
(degree of freedoms, bitwise encoded)NCOD
(additional bit code)X
(global X coordinate)Y
(global Y coordinate)Z
(global Z coordinate)
The nodes description can be found in CDBASE.CHM as shown in figure below:
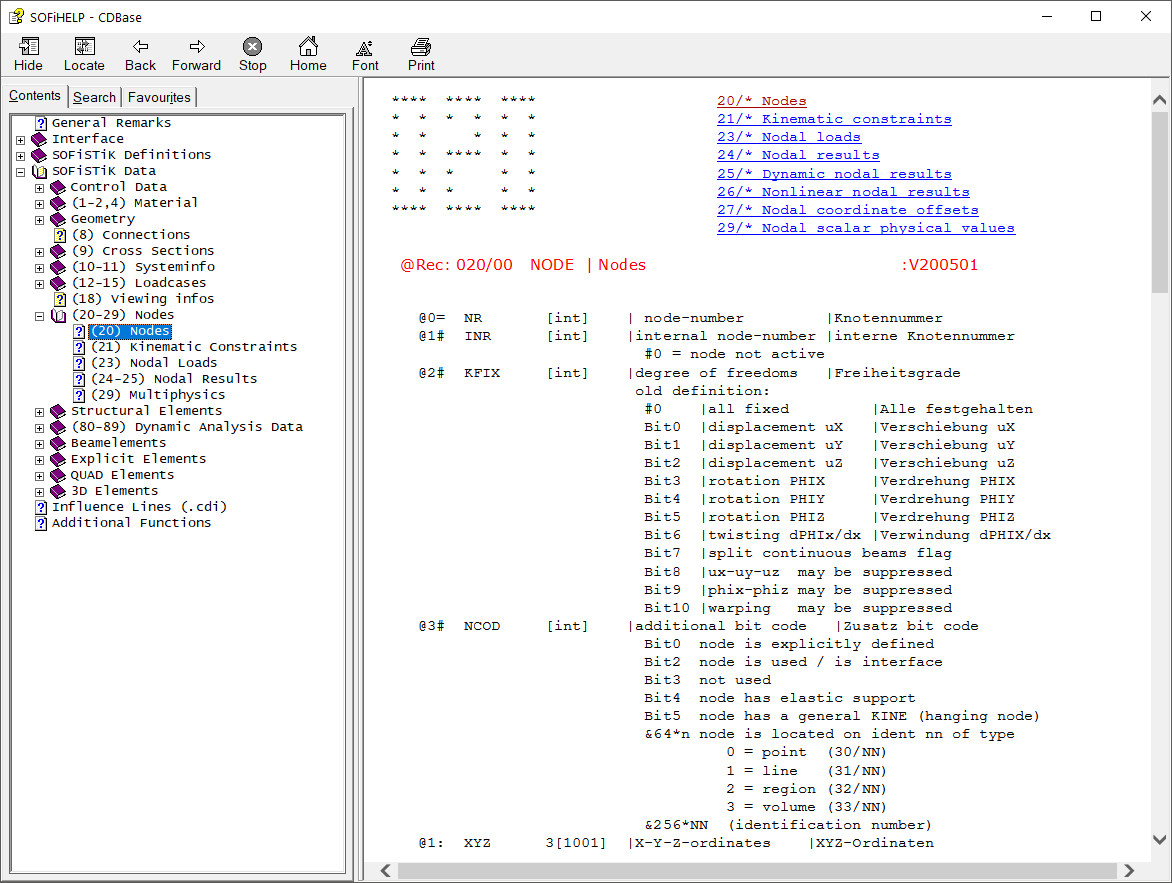
The [INT]
means that the data-type is integer. The data-type can easily be checked in the
data-type list given in the example.
Problem Solution¶
The example can be found by following:
C:\<sofistik_installation>\2020\SOFiSTiK 2020\interfaces\examples\c++\read_nodes
Here is a code example how to read the nodes from the CDB:
#include <iostream>
#include <Windows.h>
#include <string>
#include <iomanip>
// Include SOFiSTiK Libraries from :
// --> "... interfaces/examples/c++"
#include "cdbase.h"
#include "cdbtypeall.h"
#include "cdbtypecon.h"
#include "cdbtypegeo.h"
#include "cdbtypelfc.h"
#include "cdbtypemat.h"
#include "cdbtypesct.h"
#include "cdbtypesys.h"
#include "cdbtypeten.h"
using namespace std;
int main()
{
// Connect to CDB
/*
Index = 0 initialise CDBASE and open scratch data base only
Index > 0 open an existing data base with exactly tis index
(STATUS=UNKNOWN) = somehow obsolete call, use 99!
Index = 99 test if NAME is a valid database and open the base
if possible. Return with the assigned index.
If the file does not exist, it will be created.
Index = 97 open the database via pvm
Return with the assigned index.
Index = 96 open a scratch database, filename is the path to
use or NULL.
Index = 95 open in read-only mode
Index = 94 create a new data base (STATUS=NEW)
*/
int index = 99;
char* fileName = "S:\\test\\read_nodes.cdb";
int ie = sof_cdb_init(fileName, index);
if (ie < 0)
{
cout << "ERROR: Index= " << ie << " < 0 - see clib1.h for meaning of error codes" << endl;
cout << "Press any <key> to close the program";
system("pause");
return(0);
}
else if(ie == 0)
{
cout << "ERROR: Index= " << ie << " - The File is not a database" << endl;
cout << "Press any <key> to close the program";
system("pause");
return(0);
}
// Get Status
// int sof_cdb_status (int ie);
cout << "The CDB Status: " << sof_cdb_status(ie) << endl;
int datalen = sizeof(tagCDB_NODE);
int *datalenptr;
datalenptr = &datalen;
tagCDB_NODE cnode;
cout << setw(11) << "nr"
<< setw(11) << "inr"
<< setw(11) << "kfix"
<< setw(11) << "ncod"
<< setw(20) << "x"
<< setw(20) << "y"
<< setw(20) << "z" << endl;
// &cnode pass the address of the struct to pointer
while (sof_cdb_get(ie, 20, 0, &cnode, &datalen, 1) < 2)
{
cout << setw(11) << cnode.m_nr
<< setw(11) << cnode.m_inr
<< setw(11) << cnode.m_kfix
<< setw(11) << cnode.m_ncod
<< setw(20) << cnode.m_xyz[0]
<< setw(20) << cnode.m_xyz[1]
<< setw(20) << cnode.m_xyz[2]
<< endl;
}
// Close the CDB
// int sof_cdb_close (int ie);
sof_cdb_close(0);
cout << "The CDB Status after closing: " << sof_cdb_status(ie) << endl;
system("pause");
return 0;
}